Belongs to
field :user, as: :belongs_to
You will see three field types when you add a BelongsTo
association to a model.
Options
-> searchable
Turns the attach field/modal from a select
input to a searchable experience
class CourseLink < Avo::BaseResource
field :links,
as: :has_many,
searchable: true
end
WARNING
Avo uses the search feature behind the scenes, so make sure the target resource has the search_query
option configured.
# app/avo/resources/course_link_resource.rb
class CourseLinkResource < Avo::BaseResource
self.search_query = -> do
scope.ransack(id_eq: params[:q], link_cont: params[:q], m: "or").result(distinct: false)
end
end
Default
false
Possible values
true
, false
-> allow_via_detaching
Default
false
Possible values
true
, false
-> attach_scope
Default
nil
Possible values
field :user,
as: :belongs_to,
attach_scope: -> { query.non_admins }
Pass in a block where you attach scopes to the query
object. The block is executed in the AssociationScopeHost
, so follow the docs to see what variables you have access to.
-> polymorphic_as
Default
nil
Possible values
A symbol, used on the belongs_to
association with polymorphic: true
.
-> types
Default
[]
Possible values
[Post, Project, Team]
. Any array of model names.
-> polymorphic_help
Default
nil
Possible values
Any string.
-> use_resource
Default
nil
. When nothing is selected, Avo infers the resource type from the reflected association.
Possible values
PostResource
, PhotoCommentResource
, or any Avo resource class.
Overview
On the Index
and Show
views, Avo will generate a link to the associated record containing the @title
value.
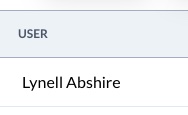

On the Edit
and New
views, Avo will generate a dropdown element with the available records where the user can change the associated model.
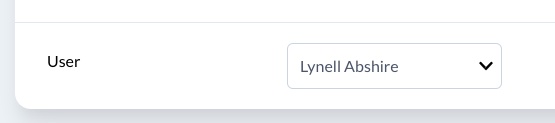
Polymorphic belongs_to
To use a polymorphic relation, you must add the polymorphic_as
and types
properties.
class CommentResource < Avo::BaseResource
self.title = :id
field :id, as: :id
field :body, as: :textarea
field :excerpt, as: :text, show_on: :index, as_description: true do |model|
ActionView::Base.full_sanitizer.sanitize(model.body).truncate 60
rescue
""
end
field :commentable, as: :belongs_to, polymorphic_as: :commentable, types: [::Post, ::Project]
end
Polymorphic help
When displaying a polymorphic association, you will see two dropdowns. One selects the polymorphic type (Post
or Project
), and one for choosing the actual record. You may want to give the user explicit information about those dropdowns using the polymorphic_help
option for the first dropdown and help
for the second.
class CommentResource < Avo::BaseResource
self.title = :id
field :id, as: :id
field :body, as: :textarea
field :excerpt, as: :text, show_on: :index, as_description: true do |model|
ActionView::Base.full_sanitizer.sanitize(model.body).truncate 60
rescue
""
end
field :reviewable,
as: :belongs_to,
polymorphic_as: :reviewable,
types: [::Post, ::Project, ::Team],
polymorphic_help: "Choose the type of record to review",
help: "Choose the record you need."
end
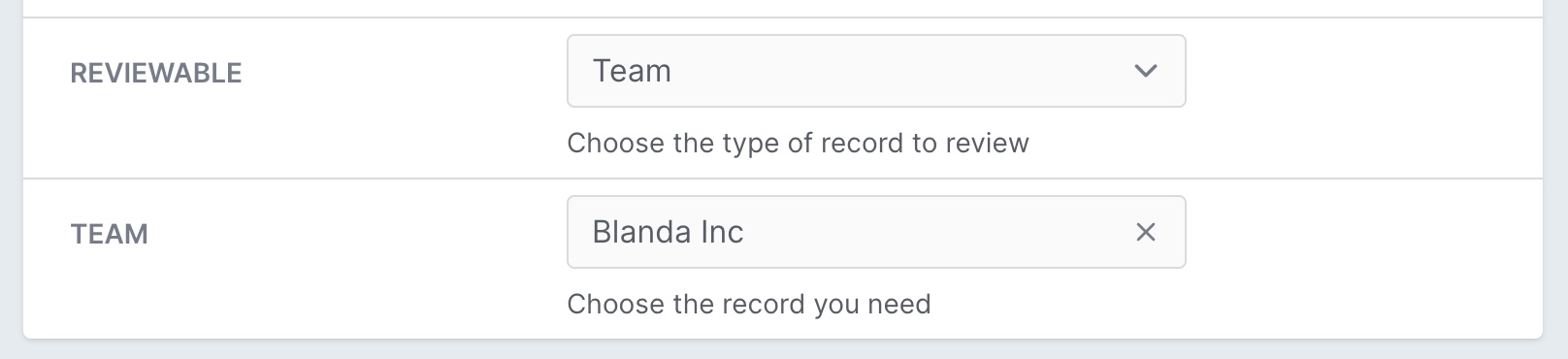
Searchable belongs_to
Watch the demo video There might be the case that you have a lot of records for the parent resource, and a simple dropdown won't cut it. This is where you can use the searchable
option to get a better search experience for that resource.
class CommentResource < Avo::BaseResource
self.title = :id
field :id, as: :id
field :body, as: :textarea
field :user, as: :belongs_to, searchable: true
end

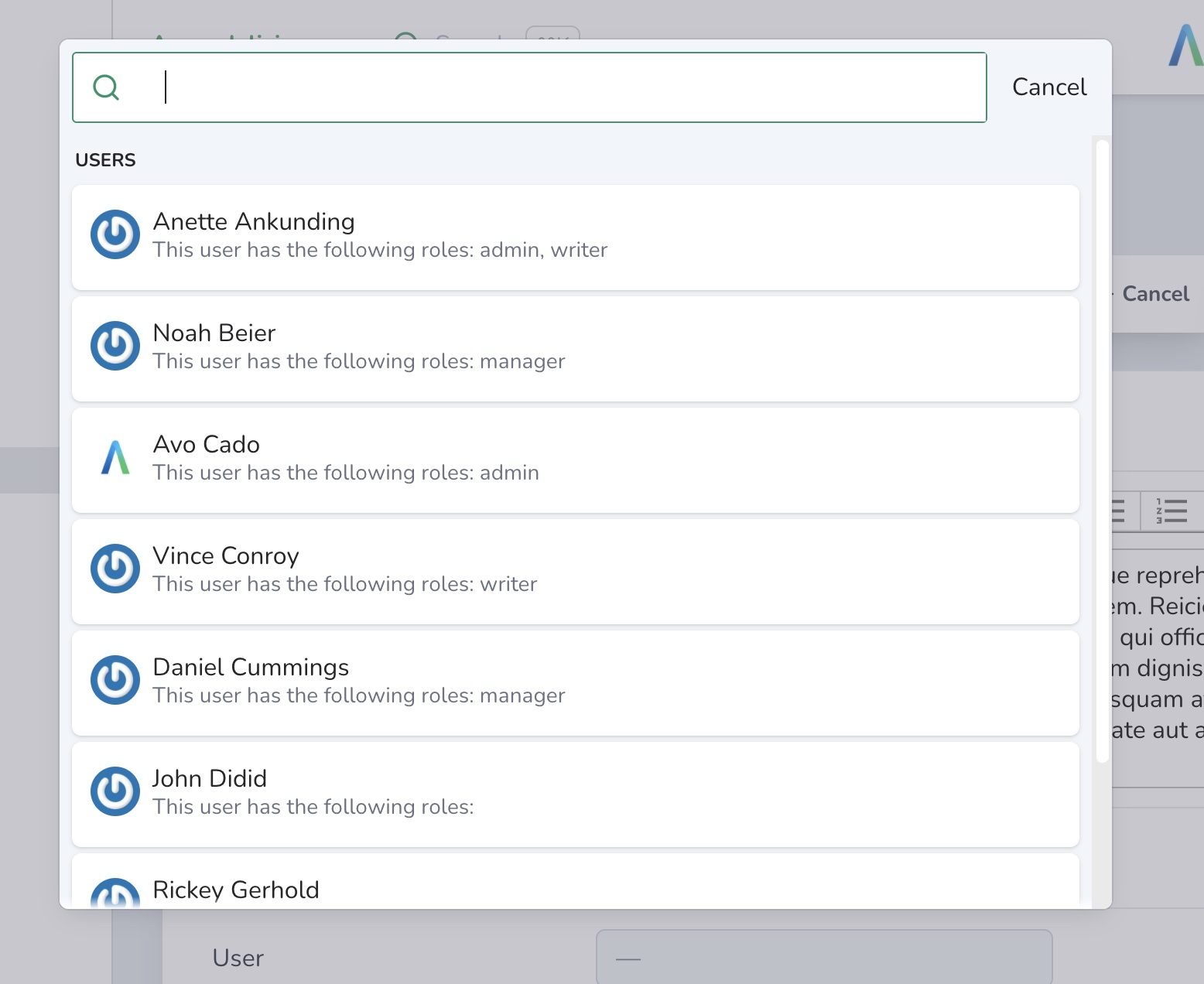
searchable
works with polymorphic
belongs_to
associations too.
class CommentResource < Avo::BaseResource
self.title = :id
field :id, as: :id
field :body, as: :textarea
field :commentable, as: :belongs_to, polymorphic_as: :commentable, types: [::Post, ::Project], searchable: true
end
INFO
Avo uses the search feature behind the scenes, so make sure the target resource has the search_query
option configured.
# app/avo/resources/post_resource.rb
class PostResource < Avo::BaseResource
self.search_query = -> do
scope.ransack(id_eq: params[:q], name_cont: params[:q], body_cont: params[:q], m: "or").result(distinct: false)
end
end
# app/avo/resources/project_resource.rb
class ProjectResource < Avo::BaseResource
self.search_query = -> do
scope.ransack(id_eq: params[:q], name_cont: params[:q], country_cont: params[:q], m: "or").result(distinct: false)
end
end
Belongs to attach scope
Watch the demo videoWhen you edit a record that has a belongs_to
association, on the edit screen, you will have a list of records from which you can choose a record to associate with.
For example, a Post
belongs to a User
. So on the post edit screen, you will have a dropdown (or a search field if it's searchable) with all the available users. But that's not ideal. For example, maybe you don't want to show all the users in your app but only those who are not admins.
You can use the attach_scope
option to keep only the users you need in the belongs_to
dropdown field.
You have access to the query
that you can alter and return it and the parent
object, which is the actual record where you want to assign the association (the true Post
in the below example).
# app/models/user.rb
class User < ApplicationRecord
scope :non_admins, -> { where "(roles->>'admin')::boolean != true" }
end
# app/avo/resources/post_resource.rb
class PostResource < Avo::BaseResource
field :user, as: :belongs_to, attach_scope: -> { query.non_admins }
end
For scenarios where you need to add a record associated with that resource (you create a Post
through a Category
), the parent
is unavailable (the Post
is not persisted in the database). Therefore, Avo makes the parent
an instantiated object with its parent populated (a Post
with the category_id
populated with the parent Category
from which you started the creation process) so you can better scope out the data (you know from which Category
it was initiated).
Allow detaching via the association
When you visit a record through an association, that belongs_to
field is disabled. There might be cases where you'd like that field not to be disabled and allow your users to change that association.
You can instruct Avo to keep that field enabled in this scenario using allow_via_detaching
.
class CommentResource < Avo::BaseResource
self.title = :id
field :id, as: :id
field :body, as: :textarea
field :commentable,
as: :belongs_to,
polymorphic_as: :commentable,
types: [::Post, ::Project],
allow_via_detaching: true
end